1. Using the If then statement
if-then syntax
One of the most used statements in scripts is the If ~ then statement. In this syntax, if the execution result of the commands defined after the if (exit code 0) is normally executed, then the command in the then clause is executed. It is not performed. The basic syntax is as follows.
- Usage syntax
if command
then
commands
fi
if command; then
commands
fi
So, let's create an example that lists the user's bash shell files if there is a corresponding user in /etc/passwd.
$touch test10
$gedit test10
#! /bin/bash
#if then statement Executing multiple commands
testuser=hyowon
if grep $testuser /etc/passwd
then
echo "The bash files for user $testuser are:"
ls -a /home/$testuser/.b*
fi
#
and if you run
$ ./test10
hyowon:x:1000:1000:hyowon,,,:/home/hyowon:/bin/bash
The bash files for user hyowon are:
/home/hyowon/.bash_history /home/hyowon/.bash_logout /home/hyowon/.bashrc
You can see that the then statement is executed normally. However, if you test with a value that does not exist in the passwd file in testuser, there is no output as shown below.
$ ./test10
$
elseif syntax
You can think of the elseif statement as an extension of the if statement. I think that the fact that you can use multiple conditional ifs is better than if statements. And the usage syntax is as follows. Like the if statement, when the exit code of the elseif statement is 0 (when the command execution result is normal), the then statement is executed.
if command1
then
commands
elif command2
then
more commands
fi
2. Using the Test command
In the if-then-elseif statement, the exit code determines whether or not to execute the command, but there may be cases where you want to execute the then command as the result of the conditional clause comparison. As for the usage syntax, you can give the condition to be tested after the test command as shown below. The test command checks the following conditional clause and returns an exit code of 0 if true and 1 if false. The disadvantage of test command is that test command cannot handle floating point. If you use it to handle floating point like the bc command, you won't get the desired result because test doesn't support floating point. Also, instead of the test command, it is possible to use conditional clauses by grouping them with brackets.
The conditions that can be compared with the test are the following three cases.
- number comparison
- string comparison
- Compare files
construction
test condition or if [ condition ]
==> A space must be inserted between the bracket and the Condition.
then
commands
fi
Numeric comparison example
$touch test11
$gedit test11
#Number and Variable Comparison Example
val1=15
val2=20
if [ $val1 -gt 5 ]
then
echo "The test value $val1 is greater than 5"
fi
if [ $val1 -eq $val2 ]
then
echo "The values are equal"
else
echo "The values are different"
fi
Then let's run it.
$ ./test11
The test value 15 is greater than 5
The values are different
The table below is the comparison operator table.
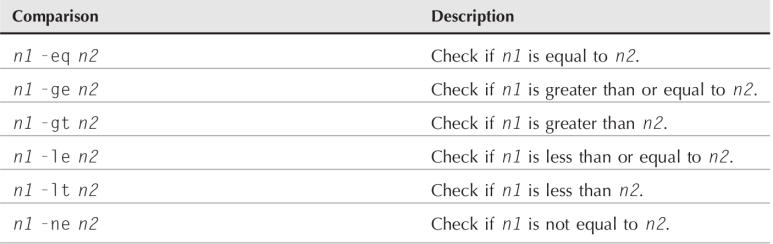
String comparison
The test command can also compare strings. Let's create a simple example as below.
#! /bin/bash
# test string comparison example
tempuser=hyowon
if [ $USER = $tempuser ]
==> Compare whether the currently logged in user and tempuser name are the same
then
echo "Welcome $tempuser"
fi
#
let's run
$ ./test13
Welcome hyowon
Below are the operators that can be used for string comparison.

string order
When performing string comparison, you must pay attention to the following two points.
1) A backslash must be entered before the > and < comparison operators so that it is not recognized as a redirect.
2) The result of >, < is not the same as sort naming. The comparison operator does not compare in ASCII code, but sort sorts in the order of system location language setting.
Below is an example.
$ cat test9b
#!/bin/bash
# testing string sort order
val1=Testing
val2=testing
if [ $val1 \> $val2 ]
then
echo "$val1 is greater than $val2"
else
echo "$val1 is less than $val2"
fi
$ ./test9b
Testing is less than testing
$ sort testfile
testing
Testing
$
String length comparison
Let's create an example string length comparison.
#! /bin/bash
#String length comparison example
val1=test
val2=' '
if [ -n $val1 ]
then
echo "The string 'val1' is not empty"
else
echo "The string '$val1' is empty"
fi
if [ -z $val2 ]
then
echo "The string ’$val2’ is empty"
else
echo "The string ’$val2’ is not empty"
fi
if [ -z $val3 ]
then
echo "The string ’$val3’ is empty"
else
echo "The string ’$val3’ is not empty"
fi
let's run
$ ./test14
The string 'test' is not empty
The string ’ ’ is empty
The string ’’ is empty
The table below shows operators that compare the lengths of strings.
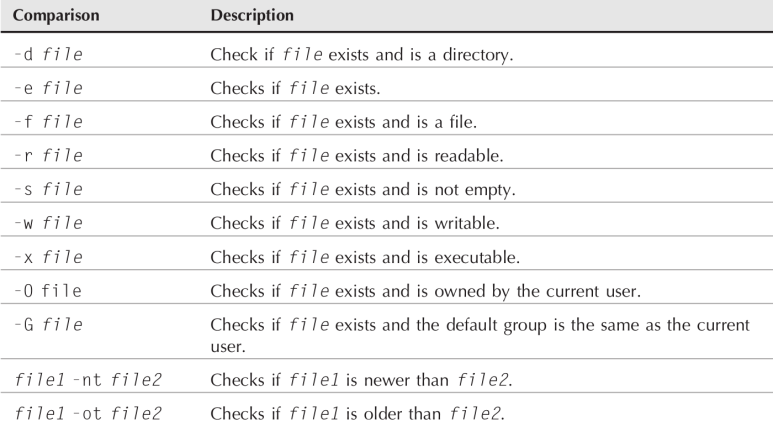
Compare files
The test command can also compare files. First, let's look at an example of directory comparison. The -d option checks whether a directory exists or not.
$touch test15
$gedit test15
#! /bin/bash
#example
if [ -d $HOME ]
then
echo "Your Home directory exists"
cd $HOME
ls
else
echo "Threr's problem with your HOME directory"
fi
let's run
$ ./test15
Your Home directory exists
1234.mkv
13826761_MotionElements_energetic-action-sport-rock-30sec-powerful-aggressive-background-music_preview-2.mp3
13826761_MotionElements_energetic-action-sport-rock-30sec-powerful-aggressive-background-music_preview.mp3
2020-10-10 15-25-47.mkv
NPKI
Steam
VirtualBox VMs
age.txt
deja-dup
dir.Iu6oW4
dir.d03tYD
This time, we'll write a script that examines directories and files, creates them if they don't exist, and adds information to them.
#! /bin/bash
#After checking for the existence of a file under the directory, add it if it does not exist
if [ -e $HOME ]
then
echo "Direcroty exists. let's check file"
#checking file
if [ -e $HOME/testing ]
then
echo " file exists, append data to it"
date >> $HOME/testing
else
echo "creating file"
date > $HOME/testing
fi
else
echo "no Home directory"
fi
let's run
$ ./test16
Direcroty exists. let's check file
creating file
$ ./test16
Direcroty exists. let's check file
file exists, append data to it
$
The --e option tells whether files and directories exist. You should use the -f option to check if it is a file only. Let's make an example
#!/bin/bash
# check if a file
if [ -e $HOME ]
then
echo "The object exists, is it a file?"
if [ -f $HOME ]
then
echo "Yes, it’s a file!"
else
echo "No, it’s not a file!"
if [ -f $HOME/.bash history ]
then
echo "But this is a file!"
fi
fi
else
echo "Sorry, the object doesn’t exist"
fi
let's run
$ ./test17
The object exists, is it a file?
No, it’s not a file!
But this is a file!
$
File read permission check example
Let's create an example that checks if a file exists, and then checks if it has read permission.
.
#!/bin/bash
#Check if the file is readable
pwfile=/etc/shadow
#First check if the file exists
if [ -f $pwfile ]
then
# Check if it is readable
if [ -r $pwfile ]
then
tail $pwfile
else
echo "Sorry, I’m unable to read the $pwfile file"
fi
else
echo "Sorry, the file $file doesn’t exist"
fi
#
let's run
$ ./test18
Sorry, I’m unable to read the /etc/shadow file
$ ./test18
Check for empty files
The -s option checks whether the file is empty or not and returns the result. Let's make an example.
#!/bin/bash
# testing if a file is empty
file=t15test ==>Enter the value to be used as the file name in the file variable.
touch $file ==> Create a file with input values
if [ -s $file ] ==>Check if the file is empty
then
echo "The $file file exists and has data in it" ==> This statement will be executed.
else
echo "The $file exists and is empty"
fi
date > $file ===> Puts the result of date into the file value.
if [ -s $file ] ==>Checking the file is not empty
then
echo "The $file file has data in it"
else
echo "The $file is still empty" ==>
fi
#
let's run
$./test15
The t15test exists and is empty
The t15test file has data in it
$
Check file write permission
The -w option checks whether the file has write permission. Let's make an example
#!/bin/bash
# checking if a file is writeable
logfile=$HOME/t16test
touch $logfile
chmod u-w $logfile ==> Remove write permission.
now=`date +%Y%m%d-%H%M`
==> In the now variable, the execution result of date is formatted and saved.
if [ -w $logfile ]
==> If the file exists and you have write permission, then execute the then statement
then
echo "The program ran at: $now" > $logfile
echo "The fist attempt succeeded"
else
echo "The first attempt failed"
fi
chmod u+w $logfile
if [ -w $logfile ]
then
echo "The program ran at: $now" > $logfile
echo "The second attempt succeeded"
else
echo "The second attempt failed"
fi
let's run
$ ./test16
The first attempt failed
The second attempt succeeded
$ cat $HOME/t16test
The program ran at: 20070930-1602
$
Checking the execute permission of a file
$ cat test17
#!/bin/bash
# testing file execution
if [ -x test16 ]
then
echo "You can run the script:"
else
echo "Sorry, you are unable to execute the script"
fi
let's run
$ ./test17
You can run the script:
Checking Ownership of a File
-0 is an option to check if the file's owner is the current user. Let's make an example
#!/bin/bash
# check file ownsership
if [ -O /etc/passwd ]
then
echo "You’re the owner of the /etc/passwd file"
else
echo "Sorry, you’re not the owner of the /etc/passwd file"
fi
$ ./test18
Sorry, you’re not the owner of the /etc/passwd file
$ su
Password:
# ./test18
You’re the owner of the /etc/passwd file
#
Check the group's default group and make sure it's the same as the user's group.
#!/bin/bash
# check file group test
if [ -G $HOME/testing ]
then
echo "You’re in the same group as the file"
else
echo "The file is not owned by your group"
fi
$ ls -l $HOME/testing
-rw-rw-r-- 1 rich rich 58 2007-09-30 15:51 /home/rich/testing
$ ./test19
You’re in the same group as the file
$ chgrp sharing $HOME/testing
$ ./test19
The file is not owned by your group
$
A script to compare the creation date of two files.
It is often used as a way to compare the installation date of a file and prevent installation when the file to be installed is an outdated version.
file1 -nt file2 : run if file1 is not older than file2 file1 -ot file2 : run if file1 is older than file2
file1 -nt file2 : run if file1 is not older than file2 file1
#!/bin/bash
# testing file dates
if [ ./test19 -nt ./test18 ]
then
echo "The test19 file is newer than test18"
else
echo "The test18 file is newer than test19"
fi
if [ ./test17 -ot ./test19 ]
then
echo "The test17 file is older than the test19 file"
fi
let's run
$ ./test20
The test19 file is newer than test18
The test17 file is older than the test19 file
$ ls -l test17 test18 test19
-rwxrw-r-- 1 rich rich 167 2007-09-30 16:31 test17
-rwxrw-r-- 1 rich rich 185 2007-09-30 17:46 test18
-rwxrw-r-- 1 rich rich 167 2007-09-30 17:50 test19
$
3. Compare multiple conditional clauses
It can be used by attaching multiple conditional clauses. That way, you can do an and operation or an or operation.
■ [ condition1 ] && [ condition2 ] ==> condition 1 and condition 2 must be true then statement is executed
■ [ condition1 ] || [ condition2 ] ==> If either condition 1 or condition 2 is true, then statement is executed
Example
$ cat test22
#!/bin/bash
# testing compound comparisons
if [ -d $HOME ] && [ -w $HOME/testing ]
then
echo "The file exists and you can write to it"
else
echo "I can’t write to the file"
fi
$ ./test22
I can’t write to the file
$ touch $HOME/testing
$ ./test22
The file exists and you can write to it
$
4. Advanced If-Then function
Among the if-then statements, there are two recently added functions
.
■ Use of parentheses for mathematical expressions
■ Using double brackets for string handling
Script example using double brackets
#!/bin/bash
# using double parenthesis
val1=10
if (( $val1 ** 2 > 90 ))
then
(( val2 = $val1 ** 2 ))
echo "The square of $val1 is $val2"
fi
$ ./test23
The square of 10 is 100
$
The types of operators using double parentheses are as follows.

Scripts with double brackets
#!/bin/bash
# using pattern matching
if [[ $USER == r* ]]
then
echo "Hello $USER"
else
echo "Sorry, I don’t know you"
fi
$ ./test24
Hello rich
$
5. Case command
In case, the command to be executed in each case can be designated separately.
#!/bin/bash
# looking for a possible value
if [ $USER = "rich" ]
then
echo "Welcome $USER"
echo "Please enjoy your visit"
elif [ $USER = barbara ]
then
echo "Welcome $USER"
echo "Please enjoy your visit"
elif [ $USER = testing ]
then
echo "Special testing account"
elif [ $USER = jessica ]
then
echo "Don’t forget to logout when you’re done"
else
echo "Sorry, you’re not allowed here"
fi
$ ./test25
Welcome rich
Please enjoy your visit
$
'Shell Script' 카테고리의 다른 글
9. User data input processing (1) | 2022.08.13 |
---|---|
8 Detailed Structed Shell Command (1) | 2022.08.12 |
6. Basic Shell Script writing (3) | 2022.08.11 |
5 Linux File Permission (2) | 2022.08.11 |
4 Environment variables (1) | 2022.08.11 |